Spring Bootcamp
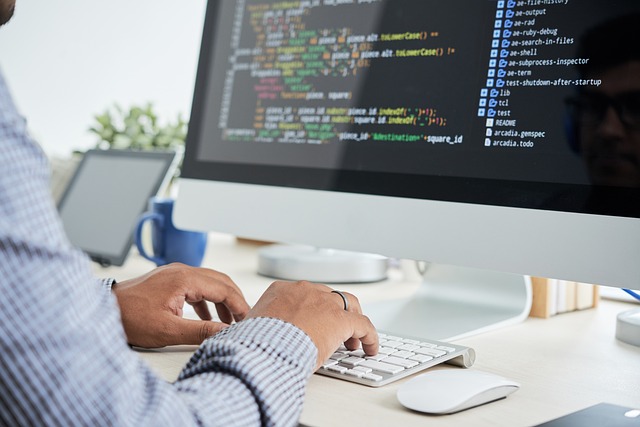
Objectives
- Build a functional Java/Spring back-end applicationÂ
- Basic CRUD operations connected to a database
- Knowledge to further application enhancements.
Module 1: Introduction to Java Spring Framework
Session 1: Overview of Java Spring
Objectives:Â
- Familiarize with the Spring framework and its ecosystem.
Topics:
- Introduction to Spring and its modules
- Benefits of using Spring for back-end development
- Setting up a Spring Boot project
Project Task:Â
- Create a new Spring Boot project setup and initialize a simple REST controller.
Session 2: Spring Boot Basics
Objectives:Â
- Understand the basics of Spring Boot and its components.
Topics:
- Spring Boot starters and dependencies
- Application properties and configuration
- Running and testing a Spring Boot application
Project Task:Â
- Add a simple endpoint to the REST controller and test it.
Module 2: Building a RESTful Web Service
Session 3: Creating RESTful APIs with Spring Boot
Objectives:Â
- Learn to create RESTful web services.
Topics:
- Understanding REST principles
- Creating REST endpoints using Spring MVC
- HTTP methods and status codes
Project Task:Â
- Implement CRUD operations for a basic entity (e.g., Product) and create corresponding endpoints.
Session 4: Connecting to a Database
Objectives:Â
- Connect a Spring Boot application to a relational database.
Topics:
- Introduction to Spring Data JPA
- Configuring a datasource and entity mapping
- CRUD operations using Spring Data repositories
Project Task:Â
- Configure a database connection and create an entity repository for the project.
Module 3: Data Persistence and Management
Session 5: Working with Spring Data JPA
Objectives:Â
- Perform data persistence and retrieval.
Topics:
- JPA entities and relationships
- Query methods and custom queries
- Handling transactions and exceptions
Project Task:Â
- Implement data persistence for the project entity with basic CRUD operations.
Session 6: Validation and Error Handling
- Objectives: Implement validation and error handling mechanisms.
Topics:
- Input validation using Hibernate Validator
- Custom error responses
- Exception handling in Spring Boot
Project Task:Â
- Add validation to the project entity and handle errors gracefully.
Module 4: Security and Authentication
Session 7: Securing Spring Boot Applications
Objectives:Â
- Implement security features in a Spring Boot application.
Topics:
- Introduction to Spring Security
- Configuring authentication and authorization
- JWT for securing REST APIs
Project Task:Â
- Secure the project endpoints with Spring Security and JWT.
Session 8: User Authentication and Authorization
Objectives:Â
- Manage user authentication and authorization.
Topics:
- Creating user entities and roles
- Implementing login and registration endpoints
- Securing endpoints based on roles
Project Task:Â
- Add user authentication and authorization to the project.
Module 5: Advanced Spring Boot Concepts
Session 9: Consuming External APIs
Objectives:Â
- Integrate external APIs with Spring Boot.
Topics:
- RestTemplate and WebClient
- Making HTTP requests to external services
- Handling responses and errors
Project Task:Â
- Consume an external API and integrate it into the project.
Session 10: Spring Boot Profiles and Configuration Management
Objectives:Â
- Manage application configurations across different environments.
Topics:
- Using Spring Boot profiles
- Externalized configuration
- Best practices for configuration management
Project Task:Â
- Set up different profiles for the project (e.g., development, production).
Module 6: Completing the Project and Further Learning
Session 11: Integrating and Testing the Application
Objectives:Â
- Perform integration and testing of the application.
Topics:
- Unit and integration testing with Spring Boot
- Mocking dependencies
- Using Testcontainers for integration tests
Project Task:Â
- Write tests for the project endpoints and perform integration testing.
Session 12: Completing the Final Project and Next Steps
Objectives:Â
- Finalize the project and suggest further learning paths.
Topics:
- Completing the back-end application
- Code review and best practices
- Advanced topics for further learning: Microservices with Spring Cloud, Reactive Programming, Spring WebFlux
Project Task:Â
- Final project demo and presentation.
- Categories
- Applications Engineering, IT, Learn, and Software Development