Spring Microservices
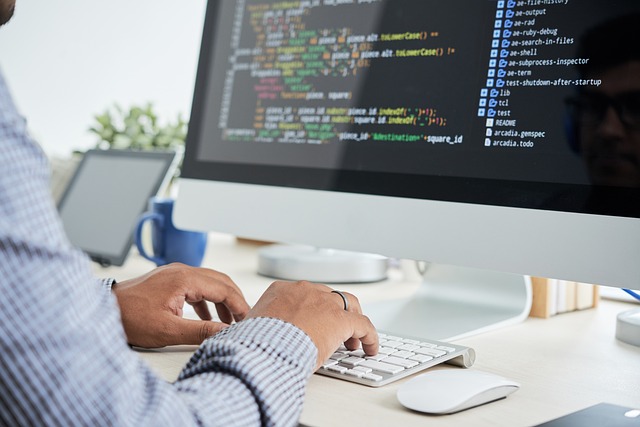
Objectives
- Understand networking fundamentals.
- Practical experience with popular network protocols.
- Design and manage networks for application development.
Module 1: Introduction to Microservices Architecture
Session 1: Overview of Microservices
Objectives:Â
Understanding the basics of Microservices and their benefits.
Topics:
- Microservices vs Monolithic architecture
- Benefits and challenges of Microservices
- Case studies of successful Microservices implementations
Exercises: - Compare and contrast different architectures; Identify services in a monolithic application that can be split into microservices.
Session 2: Spring Framework Basics
Objectives:Â
Familiarization with Spring framework and its components.
Topics:
- Introduction to Spring Boot
- Key components: Spring MVC, Spring Data, Spring Security
- Setting up a Spring Boot project
Exercises: - Create a simple Spring Boot application.
Module 2: Designing Microservices
Session 3: Decomposing the Application
Objectives:Â
Learn how to decompose a monolithic application into microservices.
Topics:
- Identifying boundaries and services
- Designing APIs for communication
- Best practices for service decomposition
Exercises: - Break down a sample monolithic application into microservices.
Session 4: Data Management in Microservices
Objectives:Â
Understanding data management strategies.
Topics:
- Database per service pattern
- Managing transactions across services
- Event sourcing and CQRS
Exercises: - Implement database per service in the sample project.
Module 3: Building Microservices
Session 5: Creating and Deploying Microservices with Spring Boot
Objectives:Â
Hands-on creation of microservices.
Topics:
- Building and running microservices with Spring Boot
- Configuring Spring Boot for different environments
- Deploying microservices to a server
Exercises: - Create and deploy your first microservice.
Session 6: Service Discovery and Load Balancing
Objectives:Â
Implement service discovery and load balancing.
Topics:
- Introduction to Netflix Eureka for service discovery
- Load balancing with Spring Cloud and Ribbon
- Configuring and using Eureka and Ribbon
Exercises: - Implement service discovery and load balancing for the project.
Module 4: Advanced Microservices Concepts
Session 7: Communication Between Microservices
Objectives:Â
Learn inter-service communication strategies.
Topics:
- RESTful communication
- Asynchronous communication using messaging (RabbitMQ/Kafka)
- Handling communication failures
Exercises: - Implement REST and messaging communication in the project.
Session 8: Securing Microservices
Objectives:Â
Implement security measures in microservices.
Topics:
- Securing APIs with OAuth2 and JWT
- Implementing security in Spring Boot
- Managing user authentication and authorization
Exercises: - Secure your microservices project.
Module 5: Monitoring and Maintenance
Session 9: Monitoring and Logging
Objectives:Â
Set up monitoring and logging for microservices.
Topics:
- Introduction to monitoring tools (Prometheus, Grafana)
- Centralized logging with ELK stack
- Configuring monitoring and logging in Spring Boot
Exercises: - Implement monitoring and logging for the project.
Session 10: Scaling and Resilience
Objectives:Â
Understand how to scale and ensure resilience.
Topics:
- Scaling microservices
- Implementing resilience patterns (circuit breaker, retries)
- Using Spring Cloud Netflix for resilience
Exercises: - Add resilience and scaling to the project.
Module 6: Bringing It All Together
Session 11: Integrating and Testing Microservices
Objectives:Â
Integrate and test the entire microservices application.
Topics:
- Integration testing
- End-to-end testing strategies
- Continuous integration and deployment (CI/CD)
Exercises: - Perform integration and testing on the project.
Session 12: Completing the Project and Further Learning
Objectives:Â
Finalize the project and discuss further learning paths.
Topics:
- Completing the hands-on project
- Code review and best practices
- Advanced topics for further learning: Kubernetes, Service Meshes, Advanced Security
Exercises: - Final project demo and presentation.
- Categories
- Applications Engineering, IT, and Learn